Introduction
The release of React Navigation brought many changes and improvements to the library. But with great progress comes great refactoring. In this guide, we will walk through setting up an authentication flow on an Expo React Native app using React’s Context and Hooks and the new React Navigation 5.0.
For the sake of simplicity, in this guide, I will assume that you know how to add AWS Cognito with amplify on your project. Otherwise there are many great guides out there to get you started. You can also check here. The approach you read in this guide can remain implemented using a different user identity service.
What is React Navigation 5?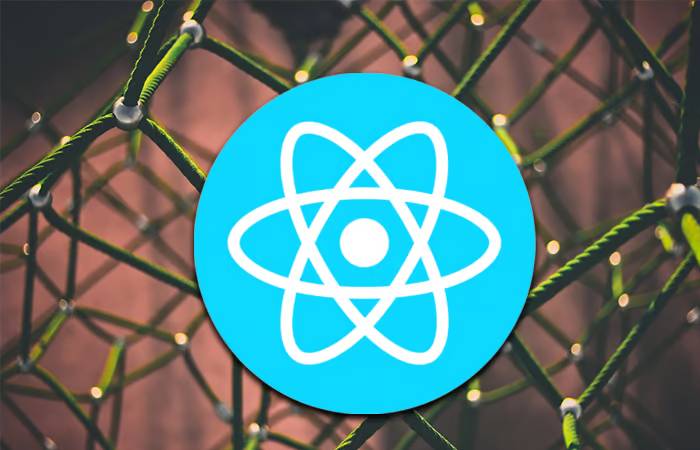
React Navigation 5.0 – A new way to navigate two years ago, we published the first stable version of React Navigation. The library has been actively developed throughout this time by adding many new features and bug fixes.
The essence of React Navigation was that it was a project that was to become a project of individual programmers adapting it to their requirements and a community as a whole, hence the emphasis on versatility, extensibility, and the tendency to reconsider the assumptions if there were such needs. Thanks to this, the Library has undergone a metamorphosis of incremental and completely reorganized shapes.
Highlights
In previous versions of React Navigation, we used to configure the navigator statically using creating navigator functions and static navigation options. In React Navigation v5, all configurations happen inside a component and are dynamic.
Example
- function App() {
- return (
- <Stack.Navigator initialRouteName=”home”>
- <Stack.Screen name=”settings” component={Settings} />
- <Stack.Screen
- name=”profile”
- component={Profile}
- options={{ title: ‘John Doe’ }}
- />
- </Stack.Navigator>
- );}
This means we have access to props, state and context and can dynamically change the configuration for the navigator! We want to stress that this is the most crucial change. This seems to be just a difference in the API. It required reconsidering many of the assumptions made in React Navigation v5 during the development of previous versions.
The static API, known for earlier versions, may seem a more accessible and obvious choice. In the current version, the navigation configuration is consistent with all patterns in the React community. This made it necessary to rewrite the library’s core, which allowed us to create some improvements not only in this respect.
New Hooks
Hooks are great for stateful logic and code organization. Now we have several angles for everyday use cases:
- Use Navigation
- Use Route
- If Use Navigation State
- Use Focus Effect
- Use Is Focused
- And also, if Use Scroll To Top
Update Options from the Component
We’ve added a new set Options method on the navigation prop to make configuring screen navigation options more intuitive than the static navigation options predecessor. And also,It lets us easily set screen options based on support, state or context without messing with params. Instead of using fixed options, we can call it anytime to configure the screen.
A Complete Guide to React Navigation 5
Developing mobile applications involves moving parts from styling, functionality, authentication, and session persistence to navigation. This article aims to arm you with the necessary skills and knowledge to build complex and straightforward navigation systems in React Native-powered apps using React Navigation V5.
React Navigation is a library consisting of components that make building and connecting screens in React Native easy. And also, It is crucial to understand how navigation in mobile applications works and what significant differences exist between mobile-based navigation and web-based navigation.
Types in React Navigation 5
- Drawer navigation
This consists of patterns in navigation that require you to use a drawer from the left (sometimes right) side for navigating between and through screens. Typically, it consists of links that provide a gateway to moving between screens. It’s similar to sidebars in a web-based application. And also, Twitter is a popular application that makes use of drawer navigation.
- Tab navigation
This is the most common form of navigation in most mobile applications. Navigation is possible through tab-based components. It consists of a bottom and top tabs. A popular application that makes use of tab-based navigation is Instagram. You use the home screen for bottom navigation and the profile screen for full tab navigation.
- Stack navigation
The transition between screens is made possible in the form of stacks. Various screens are stacked on top of each other, and movement between screens involves replacing one screen with another. And also, a popular application that uses stack navigation is the WhatsApp chat screen.
Most times, mobile applications use all types of navigators to build the various screens and functionalities of the application. These are called nesting navigators.
This article covers how to create these navigations one after the other. Then, we’ll proceed to combine them through nesting.
- Prerequisites
- Nodejs LTS release or greater
- Expo (installation below)
- And also, Watchman for Mac users
For this tutorial, we’ll be making use of Expo. Expo makes developing mobile applications using React Native very easy, and also. There are loads of other benefits, so you should check the documentation here.
Example of React Native Navigation 5.0
You can link to various pages using an anchor <a> tag in a web browser. When the user clicks on a link, the URL is pushed to the browser history stack, and also. When the user clicks on the back button, and also. And also, the browser displays the item from the top of the history stack, so the current page is now the previously visited page.
React Native doesn’t have a built-in concept of a global history stack like a web browser does; this is where React Navigation comes in handy. And also, React Navigation’s stack navigator allows your app to transition between screens and maintain navigation history.
Announcing React Navigation 5.0
This version is still in alpha and won’t be stable until sometime in 2020! More adventurous users are already using it in production, but it’s not for everyone. That said, we would love your feedback. And also, it will help us ship the stable release sooner, so please give it a try and even try converting your app if you have the time.
We’ve been working on several improvements to React Navigation, such as improving animation performance with gesture-handler and reanimated libraries, migrating to TypeScript, etc.
Conclusion
React Navigation has long grown from a community project to becoming the most popular solution for implementing a navigation system to a React Native app. The library has seen many new features and bug fixes implemented throughout this time, solidifying its position as the standard solution for navigation in React Native apps.
Its most significant update so far was released in 2020 in the form of React Navigation v5, a long refactoring effort from the team which, aside from addressing some common pain points of past releases, also. The substantially changes the API of the library and hopes to improve its performance and ease of use.